How To Convert Fortran Program To C: Software Free Download
# # f2cpp.pl - Converts Fortran 77 code to C++ # # Unlike f2c, the output of f2cpp.pl may or may not compile, however, # only a small number of hand-tuned changes are typically needed. # The resulting code is much cleaner than f2c's output, and much closer # to the original intent of the original Fortran code.
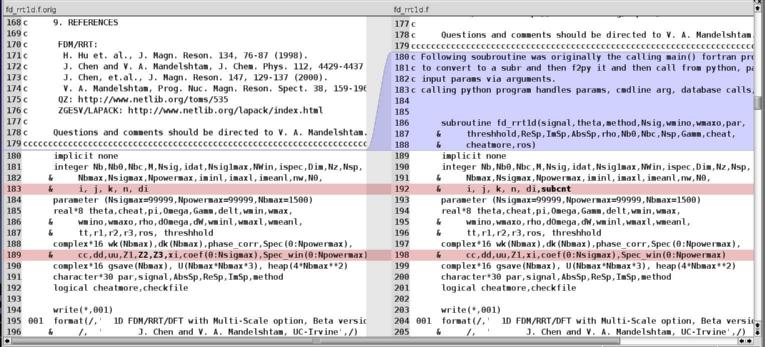
# # The resulting C++ code is very C-style; we basically only want C++ # for its std::complex type and operator overloading. # # Assumes single subroutine per file, and that there are # no lines of significance above the subroutine declaration.
MinGW - Minimalist GNU for Windows. A native Windows port of the GNU Compiler Collection (GCC). MinGW: A native Windows port of the GNU Compiler Collection (GCC), with freely distributable import libraries and header files for building native. 2,076,344 weekly downloads. Code::Blocks Icon Code::Blocks.
# # TODOs: # - Make the first step of conversion breaking up the input into chunks # delimited by subroutine boundaries. This will require full on parsing # of blocks.
# - Some declarations are generated incorrectly, like IZAMAX, whose array # argument is never an array. # - Fix by-value argument passing of expressions (currently lets through # things like (-&std::conj(tau)). # - Generate proper subroutine declarations for character arguments # (should be const char *, not char).
# - Determine const-ness of function declaration parameters through program # analysis.
As part of my Final Year Project, I need to convert some FORTRAN code into C or C++ (it doesn't matter which language as long as I can understand it, and I can understand C style languages). I have discovered f2c, a program that allegedly converts FORTRAN to C, and tried to install it, following instructions, by saving a makefile.vc file on my drive and then doing copy makefile.vc makefile nmake (here is the part of the README file about installing f2c that is included in the f2c ) To compile f2c on Linux or Unix systems, copy makefile.u to makefile, edit makefile if necessary (see the comments in it and below) and type 'make' (or maybe 'nmake', depending on your system).
To compile f2c.exe on MS Windows systems with Microsoft Visual C++, copy makefile.vc makefile nmake With other PC compilers, you may need to compile xsum.c with -DMSDOS (i.e., with MSDOS #defined). If your compiler does not understand ANSI/ISO C syntax (i.e., if you have a K&R C compiler), compile with -DKR_headers. On non-Unix systems where files have separate binary and text modes, you may need to 'make xsumr.out' rather than 'make xsum.out'. I am running x64 bit version of Windows Vista and tried 'nmake', but I get 'nmake' is not recognised as an internal or external command, operable program or batch file.
I downloaded Nmake15.exe after some searching but it doesn't work on x64 bit machines and apparently there is not version of it that does. So I downloaded the Windows SDK, after being told that would work, but it didn't change anything. Where have I gone wrong in all this, if I have, and is there any way of converting that FORTRAN code into C or C++?
The method I've been using for conversion so far is semi-automated: lots of editor and text scripts. First, collimate all the Fortran source into a single file (placing markers as appropriate to allow the files to be re-separated). Free Download Font Thai Psp 3000.
Next, convert the numeric labels to named labels (e.g. Adding an L before them for gotos and F for formats). Make the appropriate changes in the places that reference the labels. Next, mark off the label - continue combinations and the statement continuations (e.g. With a mark at the start of each line where they occur). Then re-join the label-continue and statement-continuation lines.
In an editor like vi, that would mean doing a series of (say) 1000J's, and then a massive substitute:%s/#/^V^M/g if the start of line marker is a #. Since the continuation lines were specially marked as were the do-continue combos, then they get rejoined. Ensure the if-labels and do-labels are separated (e.g.
Write a grep pattern to extract the relevant lines and then write a simple bracket-matching program to check them out). Once separated, you can then turn the do-continues into brackets. Turn the if-then-else, and dowhile/enddo, subroutine/endsub, etc.
Into brackets. Make subroutine void, pony up fake names for the basic types (e.g. Boolean, Character, Integer, Real, Double, Complex, Complex). Comments are converted over to C++ // comments; both the column 0 and!
Then you can re-prototype the functions. This is effectively a KR to ANSI-C conversion and requires a simple program to read the function headers and variable declarations to match them off. Ideally you may want to first put the variable declarations as one to a line.
This also requires an edit script and a utility. For instance a 'Double A, B, C.' Would be converted to '#Double nA nB n.' And then a utility is set up to prefix all the non-# lines with the most recent preceding # line. Parameter statements get rejoined to the type declarations and converted to 'const' statements.
Saves get converted to static declarations. Commons I haven't yet figured out a good way to work with. Now the HARD parts come next: the array dimensioning, variable in/out analysis (to determine which variables need to be passed by value or by reference).
You should get a C compiler that recognizes the reference type & or use C++. For array-redimensioning you may also need to go over to C++ which allows [] to be redefined. The in-out analysis is something I haven't yet worked through, likewise the redimensioning. But at this point, the relevant source is mostly in C or C++ at this stage. Be warned: Fortran 2010allows parallel processing, which goes beyond C and even C++ (except for their multithreaded abilities). If you programs have these in them, things will become a lot harder. You aren't real clear on this, but it sounds like you want to study the code, and in order to do that you want to convert it to C or C++.
If you want to use the code rather than study it, then I'm off-base here, and you should look for Fortran compilers. You can't find any way of translating idiomatic Fortran to idiomatic C or C++. There's subtle semantic differences between the languages, and in order to get it right the translation program needs to be very precise, and account for lots of things, and make obscure function calls, and that can be very confusing.
You will not get readable C or C++. Instead, you should learn the appropriate version of Fortran. If you understand C and C++, it shouldn't be at all hard to learn. It's just another language, and it won't have much in the way of new concepts. You need to be clear why you are doing this. Indonesian Bible Download Pdf. Is it because the functionality you need is ONLY available in some legacy FORTRAN?
I had this problem many years ago when I needed a general matrix inversion algorithm, that was only available in FORTRAN. It wasn't easy to understand - no comments and variables named like G(J). I converted it to C using f2c and it ran perfectly. But it was even harder to understand.
Two points were that FORTRAN counts from 1 and C from 0, so there were lots of i+1 and j-1. Also arguments had to be implemented by reference. Later I had to run this in Java.
Still no other algorithm, so I converted the C to Java. This was really painful. And I still didn't understand what has happening.
And after a year or two it stopped working! But, luckily, now there are several Java implementations. So if you can explain your real requirements maybe we can help. I hope that this isn't an assignment, because if so it's (IMO) a poor one. If there is some magic legacy code, suggest you try as hard as possible to find a modern equivalent. I found there is a small toolkit named fable which is dedicated to such conversion. THere is also.
Abstract from the review authors: Background In scientific computing, Fortran was the dominant implementation language throughout most of the second part of the 20th century. The many tools accumulated during this time have been difficult to integrate with modern software, which is now dominated by object-oriented languages. Results Driven by the requirements of a large-scale scientific software project, we have developed a Fortran to C++ source-to-source conversion tool named FABLE. This enables the continued development of new methods even while switching languages. We report the application of FABLE in three major projects and present detailed comparisons of Fortran and C++ runtime performances. Conclusions Our experience suggests that most Fortran 77 codes can be converted with an effort that is minor (measured in days) compared to the original development time (often measured in years). With FABLE it is possible to reuse and evolve legacy work in modern object-oriented environments, in a portable and maintainable way.
FABLE is available under a nonrestrictive open source license. In FABLE the analysis of the Fortran sources is separated from the generation of the C++ sources. Therefore parts of FABLE could be reused for other target languages.